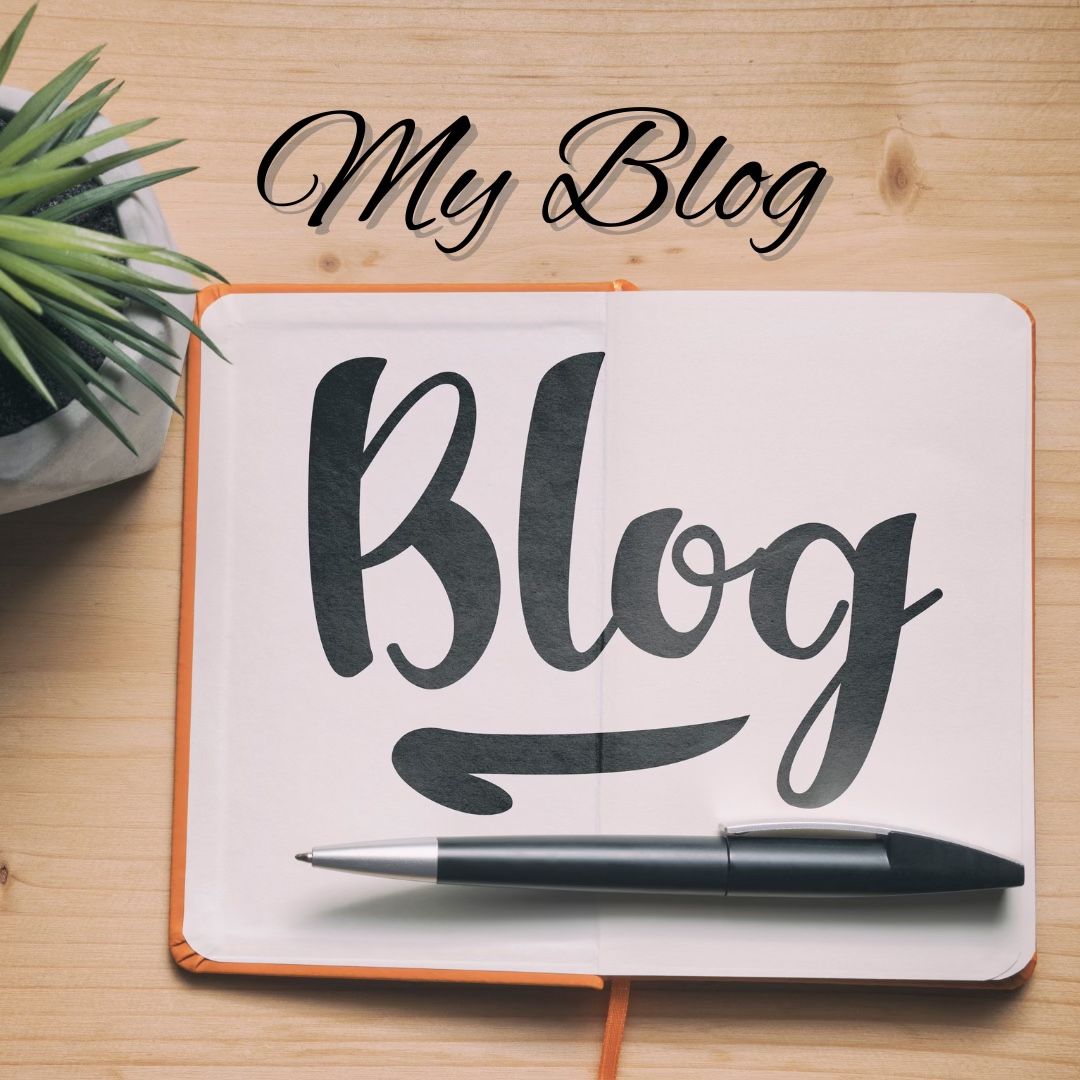
BLOG
Blog section showcases the articles I've written on various topics. This section provides a closer look into my thoughts in a textual way. You can view the details each post by clicking on the "View Details" button. It would display details of the post including the images and the description.
No blogs available.